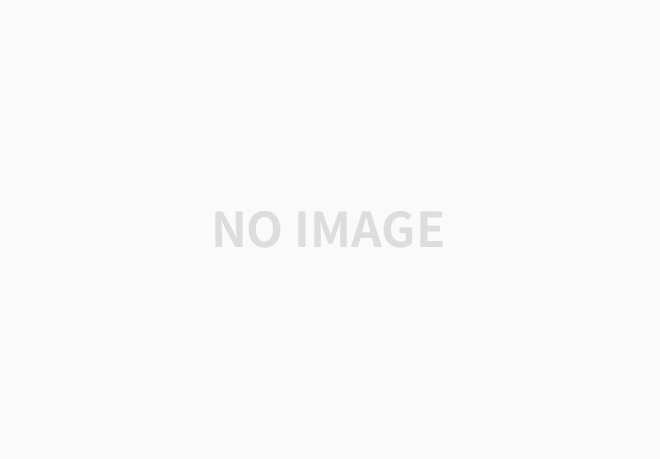
Python 과 openpyxl 을 이용해서 달력을 엑셀에 출력해 봅시다
# 달력 출력하기
from openpyxl import styles
from openpyxl.styles.borders import Border, Side
from openpyxl.styles import Font, Color, Alignment
from openpyxl import Workbook
import openpyxl
import calendar
import datetime
BORDER_NONE = None
BORDER_DASHDOT = 'dashDot'
BORDER_DASHDOTDOT = 'dashDotDot'
BORDER_DASHED = 'dashed'
BORDER_DOTTED = 'dotted'
BORDER_DOUBLE = 'double'
BORDER_HAIR = 'hair'
BORDER_MEDIUM = 'medium'
BORDER_MEDIUMDASHDOT = 'mediumDashDot'
BORDER_MEDIUMDASHDOTDOT = 'mediumDashDotDot'
BORDER_MEDIUMDASHED = 'mediumDashed'
BORDER_SLANTDASHDOT = 'slantDashDot'
BORDER_THICK = 'thick'
BORDER_THIN = 'thin'
def cell_font_style(font_color, font_size, align):
font = Font(color=font_color, size= font_size )
alignment = Alignment(horizontal="center", vertical= align)
return font, alignment
def set_title(ws, title):
style = cell_font_style("FF0000", 20, "center")
ws["A1"] = title
A1 = ws["A1"]
A1.font = style[0]
A1.alignment = style[1]
ws.row_dimensions[1].height = 60
ws.merge_cells("A1:G1")
# set_titile
def set_weeks_title(ws):
weeks = ["일", "월","화","수", "목", "금", "토"]
style = cell_font_style("0000FF", 14, "center")
for w in range(1,8):
c = ws.cell(row=3, column=w, value=weeks[w-1])
c.font = style[0]
c.alignment = style[1]
c.border = get_border(BORDER_NONE, BORDER_NONE, BORDER_THIN, BORDER_NONE)
# set_weeks
def get_border(top, right, bottom, left):
thin_border = Border(left=Side(style=left),
right=Side(style=right),
top=Side(style=top),
bottom=Side(style=bottom))
return thin_border
#get_border
def set_calendar(ws, year, month):
o = calendar.Calendar(calendar.SUNDAY)
i=4
for weeks in o.monthdays2calendar(year, month):
j=1
for week in weeks:
if (week[0] > 0):
ws.cell(row=i, column=j, value=week[0])
j = j + 1
ws.row_dimensions[i].height = 30.5
i = i + 1
#set_calendar
#create workbook
wb = Workbook()
now = datetime.datetime.now()
print(now.year, now.month, now.day, now.hour, now.minute, now.second)
year = now.year
for month in range(1,13):
if month == 1:
ws = wb.active
ws.title = "1월"
else:
ws = wb.create_sheet(str(month) + "월")
set_title(ws, str(month) + "월")
set_weeks_title(ws)
set_calendar(ws, year, month)
wb.save(filename="달력_" + str(year) +".xlsx")
엑셀에 시트별로 각각의 달력이 표시됩니다.
그밖에....
#openpyxl border style 설정하기
#openpyxl font style 설정하기
#openpyxl컬럼 머지하기
끝~
반응형
'개발 > python' 카테고리의 다른 글
[python] pyinstaller (0) | 2021.01.17 |
---|---|
[python 기초] 엑셀에 구구단 출력하기 (0) | 2021.01.05 |
파이썬 - pandas LG전자 일봉가져오기 (0) | 2020.12.29 |